Step 6) Click on 'Eclipse IDE for Java Developers' Step 7) Click on 'INSTALL' button Step 8) Click on 'LAUNCH' button. Step 9) Click on 'Launch' button. Step 10) Click on 'Create a new Java project' link. When we created our model using the UML model wizard, a UML resource was created for us, so now all that needs to be done is to serialize the contents of our model as XMI to our file on disk (i.e. To save a model using the UML editor, follow these steps: 1) Select the File Save menu item. It s that simple. Objectaid Uml Explorer Home How to install papyrus and generate java code in eclipse mars 2 uml getting started modeling in eclipse installing a maven project in eclipse version mars 2 4 5 objectaid uml explorer. Eclipse/Installation - Installing Eclipse is relatively easy, but does involve a few steps and software from at least two different.
- Objectaid Uml Explorer For Eclipse
- Download File Objectaid Uml Explorer Eclipse Tutorials
- Objectaid Uml Eclipse
- Navigation
- Main Page
- Community portal
- Current events
- Recent changes
- Random page
- Help
Ashif Page 2 Tutorial Tec. Objectaid Uml Explorer Class Diagram. Given A Folder Of Java Files Is There An Eclipse Plugin To Draw.
- Toolbox
- Page information
- Permanent link
- Printable version
- Special pages
- Related changes
- What links here
Copyright © 2004, 2014 International Business Machines Corp., CEA, and others.
Summary
This article describes how to get started with the UML2 plug-ins for Eclipse. In particular, it gives an overview of how to create models (and their contents) both programmatically and by using the sample UML editor.
Kenn Hussey and James Bruck
Last Updated: January 21, 2014
To start using UML2 (and to follow along with the example in this article), you must have Eclipse, EMF, and UML2 installed. You can either download the Modeling Tools Package or follow these steps:
- Download and run Eclipse.
- Select the Help > Install New Software… menu item.
- Select a software site to work with, e.g., Luna - http://download.eclipse.org/releases/luna.
- Expand the Modeling tree item.
- Select UML2 Extender SDK and press the Next > button.
- Review the install details and press the Next > button.
- Accept the terms of the license agreement and press the Finish button.
- Restart Eclipse when prompted to do so.
At this stage, UML2 and all dependencies should be installed.

This article will walk you through the basics of creating models using UML2. Using a simple model (the ExtendedPO2 model, shamelessly “borrowed” from the EMF “bible” [1]) as an example, we’ll look at what’s involved in creating some of the more common elements that make up a model. For each type of element, we’ll first explain the creation process using the sample UML editor and then explore how to accomplish the same thing using Java code. The ExtendedPO2 model is shown below.
Readers who don't want to follow every step of this tutorial may install a working solution from the New → Example... wizard, selecting the UML2 Example Projects → Getting Started with UML2 sample. This will be available when Enhancement 382342 is resolved and released in a UML2 build. This includes the finished model, complete source code, and a launch configuration that runs the stand-alone Java application which creates the model in the root folder of the example project.
Before getting started, you’ll need to create a simple project in your workspace. This project will serve as the container for the model that we’ll create using the UML editor. To create a simple project for this article, follow these steps:
- Select the Window > Open Perspective > Other… menu item.
- Select the Resource perspective and press the OK button.
- Select the File > New > Project... menu item.
- Select the Project wizard from the General category and press the Next > button.
- Enter a project name (e.g. “Getting Started with UML2”) and press the Finish button.
At this point your workspace should look something like this:
OK, that should be enough to get us going with the UML editor. Now, to follow along with the programmatic approach to creating models, we’ll assume that you’ve created a class (named, say, “GettingStartedWithUML2”) in which you can write some code to construct our sample model. The code snippets we’ll show assume you’ve defined the following utility methods to give the user information on the program’s status:
A static debug flag can be used to enable or disable verbose information printed to the system’s output stream. Errors will always be printed to the system’s error stream.
All right, then! In each of the following subsections, we’ll look at how to create a different kind of UML element, starting with models.
At the root of a typical UML model is a model element. It contains a (hierarchical) set of elements that together describe the physical system being modeled. To create a model using the UML editor, follow these steps:
- Select a project (e.g., Getting Started with UML2) in the Project Explorer view and select the File > New > Other... menu item.
- Select the UML Model wizard from the Example EMF Model Creation Wizards category and press the Next > button.
- Enter a file name (e.g., “ExtendedPO2.uml”) and press the Next > button.
- Select Model for the model object and press the Finish button.
- Select the Window > Show View > Properties menu item.
- Select the <Model> element in the UML editor.
- Enter a value (e.g., “epo2”) for the Name property in the Properties view.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns a model with a specified name.
First, we ask the UML factory singleton to create a model, and we set its name. Then, we output information to let the user know that the model has been successfully created. Finally, we return the model. You’ll notice most, if not all, of the code snippets in this article will follow this pattern – create the element (and set some properties on it), inform the user, and return it.
All named elements (a model is a type of named element) have a “simple” name and a qualified name. The qualified name is the “simple” name prefixed with the “simple” names of all of the named element’s containing namespaces. Note that the qualified name of a named element is only defined if all of its containing namespaces have non-empty “simple” names.
OK, let’s see this method in action. For example, we could create a model named ‘epo2’ as follows:
A package is a namespace for its members (packageable elements), and may contain other packages. A package can import either individual members of other packages, or all of the members of other packages. To create a package using the UML editor, follow these steps:
- Select a package (e.g., <Package> epo2) in the UML editor.
- Select the New Child > Nested Package > Package option from the context menu.
- Enter a value (e.g., “bar”) for the Name property in the Properties view.
We don’t actually need to create a package because our sample model doesn’t contain any &emdash; except of course for the root package (i.e., the model). That’s right: a model is a type of package.
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns a package with a specified name in a specified nesting package.
Here, instead of asking the factory to create the package for us, we’re making use of one of the factory methods in the UML2 API. In UML2, a factory method exists for every feature that can contain other elements (i.e., every containment feature). In addition, more convenient factory methods exist for commonly created types (like packages). In this case, the package has a feature (packagedElement) that can contain packageable elements, so we could obtain the Ecore class of the type of (packageable) element we want to create (i.e., Package) from the UML Ecore package singleton, and pass it to the createPackagedElement(String, EClass) factory method. Instead, we use the more convenient createNestedPackage(String) factory method which implicitly creates a package and accepts the desired package name as an argument. Behind the scenes, the package will create a nested package, set its name, and add the package to its list of packaged elements.
OK, let’s see this method in action. For example, we could create a package named ‘bar’ in nesting package ‘foo’ as follows:
A primitive type defines a predefined data type, without any relevant substructure. Primitive types used in UML™ itself include Boolean, Integer, Real, String, and UnlimitedNatural. To create a primitive type using the UML editor, follow these steps:
- Select a package (e.g., <Model> epo2) in the UML editor.
- Select the New Child > Owned Type > Primitive Type option from the context menu.
- Enter a value (e.g., “int”) for the Name property in the Properties view.
Create the remaining primitive types from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns a primitive type with a specified name in a specified package.
Here we call the createOwnedPrimitiveType(String) convenience factory method to ask the package to create a primitive type with the specified name as one of its packaged elements.
OK, let’s see this method in action. For example, we could create a primitive type named ‘int’ in model ‘epo2’ as follows:
Write code to programmatically create the remaining primitive types from the ExtendedPO2 model.
An enumeration is a kind of data type whose instances may be any of a number of user-defined enumeration literals. To create an enumeration using the UML editor, follow these steps:
- Select a package (e.g., <Model> epo2) in the UML editor.
- Select the New Child > Owned Type > Enumeration option from the context menu.
- Enter a value (e.g., “OrderStatus”) for the Name property in the Properties view.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns an enumeration with a specified name in a specified package.
Here we call the createOwnedEnumeration(String) convenience factory method to ask the package to create a primitive type with the specified name as one of its packaged elements.
Objectaid Uml Explorer For Eclipse
OK, let’s see this method in action. For example, we could create an enumeration named ‘OrderStatus’ in model ‘epo2’ as follows:
An enumeration literal is a user-defined data value for an enumeration. To create an enumeration literal using the UML editor, follow these steps:
- Select an enumeration (e.g., <Enumeration> OrderStatus) in the UML editor.
- Select the New Child > Owned Literal > Enumeration Literal option from the context menu.
- Enter a value (e.g., “Pending”) for the Name property in the Properties view.
Create the remaining enumeration literals from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns an enumeration literal with a specified name in a specified enumeration.
Here we call a createOwnedLiteral(String) convenience factory method to ask the enumeration to create an enumeration literal with the specified name as one of its owned literals.
OK, let’s see this method in action. For example, we could create an enumeration literal named ‘Pending’ in enumeration ‘OrderStatus’ as follows:
Write code to programmatically create the remaining enumeration literals from the ExtendedPO2 model.
A class is a kind of classifier whose features are attributes (some of which may represent the navigable ends of associations) and operations. To create a class using the UML editor, follow these steps:
- Select a package (e.g., <Model> epo2) in the UML editor.
- Select the New Child > Owned Type > Class option from the context menu.
- Enter a value (e.g., “Supplier”) for the Name property in the Properties view.
Create the remaining classes from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns a(n) (abstract) class with a specified name in a specified package.
Here we call the createOwnedClass(String, boolean) convenience factory method to ask the package to create a class with the specified name as one of its packaged elements, and set the isAbstract attribute of the class based on the specified boolean argument.
You may have noticed that we have been fully qualifying references to the Package and Class interfaces. This is recommended so that these types are not confused with java.lang.Class and java.lang.Package, which are imported implicitly in Java.
OK, let’s see this method in action. For example, we could create a non-abstract class named ‘Supplier’ in model ‘epo2’ as follows:
Write code to programmatically create the remaining classes from the ExtendedPO2 model.
A generalization is a taxonomic relationship between a specific classifier and a more general classifier whereby each instance of the specific classifier is also an indirect instance of, and inherits the features of, the general classifier. To create a generalization using the UML editor, follow these steps:
- Select a classifier (e.g., <Class> USAddress) in the UML editor.
- Select the New Child > Generalization > Generalization option from the context menu.
- Select a value (e.g., epo2::Address) for the General property in the Properties view.
Create the remaining generalizations from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns a generalization between specified specific and general classifiers.
Here we call a convenience factory method on the specific classifier that creates a generalization as one of its children and sets the general classifier to the specified argument.
OK, let’s see this method in action. For example, we could create a generalization between specific class ‘USAddress’ and general class ‘Address’ as follows:
Write code to programmatically create the remaining generalizations from the ExtendedPO2 model.
When a property is owned by a classifier it represents an attribute; in this case is relates an instance of the classifier to a value or set of values of the type of the attribute.
The types of Classifier that can own attributes in UML2 include Artifact, DataType, Interface, Signal, and StructuredClassifier (and their subtypes).
To create an attribute using the UML editor, follow these steps:
- Select a classifier (e.g., <Class> Address) in the UML editor.
- Select the New Child > Owned Attribute > Property option from the context menu.
- Enter a value (e.g., 'name”) for the Name property in the Properties view.
- Select a value (e.g., epo2::String) for the Type property in the Properties view.
- Enter a value (e.g., 0) for the Lower property in the Properties view.
Lower and upper values for multiplicity elements (like properties) are represented as value specifications (first-class objects) in UML™ 2.x. The default value for lower and upper bounds is 1, unless a child value specification exists, in which case its value is used. Specifying a value for the lower or upper property will create a child value specification if none exists, or update its value if one does. Note that, to be treated as a bound, the lower value must be an integer and the upper value must be an unlimited natural.
Create the remaining attributes from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns an attribute with a specified upper bound, lower bound, type, and name in a specified class.
Here we call a createOwnedAttribute(String, Type, int, int) convenience factory method to ask the class to create a property as one of its owned attributes, set the type of the attribute to the specified type, and set the lower and upper bounds of the attribute (the factory method indirectly creates a literal integer and literal unlimited natural, respectively, and sets their values to the specified integer values).
The LiteralUnlimitedNatural.UNLIMITED constant represents the unlimited value for upper bounds (-1), as it does in EMF; when setting this value in the Properties view, an asterisk (‘*’) can alternatively be specified.
OK, let’s see this method in action. For example, we could create an attribute with multiplicity 0..1 of type ‘String’ named ‘name’ in class ‘Supplier’ as follows:
Write code to programmatically create the remaining attributes from the ExtendedPO2 model.
An association specifies a semantic relationship that can occur between two or more typed instances; its ends are represented by properties, each of which is connected to the type of the end. When a property is owned by an association it represents a non-navigable end of the association, in which case the type of the property is the type of the association end.
The notion of association end navigability was separated from that of ownership in the UML™ 2.0 specification, so a property that is owned by an association isn’t necessarily non-navigable as of UML2 2.0.
To create an association using the UML editor, follow these steps:
- Select a package (e.g., <Model> epo2) in the UML editor.
- Select the New Child > Owned Type > Association option from the context menu.
- Enter a value (e.g., “A_orders_supplier”) for the Name property in the Properties view.
- Select the association (e.g., <Association> A_orders_supplier) in the UML editor.
- Select the New Child > Owned End > Property option from the context menu.
- Select a value (e.g., epo2::Supplier) for the Type property in the Properties view.
- Select a class (e.g., <Class> Supplier) in the UML editor.
- Select the New Child > Owned Attribute > Property option from the context menu.
- Select a value (e.g., Composite) for the Aggregation property in the Properties view.
- Select a value (e.g., epo2::A_orders_supplier) for the Association property in the Properties view.
- Enter a value (e.g., 'orders') for the Name property in the Properties view.
- Select a value (e.g., epo2::PurchaseOrder) for the Type property in the Properties view.
- Enter a value (e.g., 0) for the Lower property in the Properties view.
- Enter a value (e.g., *) for the Upper property in the Properties view.
Create the remaining associations from the ExtendedPO2 model using the UML editor.
At this point your workspace should look something like this:
Let’s look at how to perform the same task using Java code. The code snippet below shows a method that programmatically creates and returns an association between two specified types, with ends that have the specified upper bounds, lower bounds, role names, aggregation kinds, and navigabilities.
Here we call a convenience factory method on the first end type that creates an association (and its ends) between it and another type as one of its siblings (i.e. as a child of its package namespace) and with the specified upper bounds, lower bounds, role names, aggregation kinds, and navigabilities. The owners of the association ends (properties) are based on the specified navigabilities – navigable ends are owned by the end type if allowed, otherwise they are owned by the association; non-navigable ends are owned by the association.
The NamedElement.SEPARATOR constant represents the standard separator (::) used in qualified names.
OK, let’s see this method in action. For example, we could create a unidirectional composition (composite association) between classes ‘Supplier’ and ‘PurchaseOrder’ in model ‘epo2’ as follows:
Write code to programmatically create the remaining associations from the ExtendedPO2 model.
Now that we’ve spent all this time creating a model, we’d better save our work. When we created our model using the UML model wizard, a UML resource was created for us, so now all that needs to be done is to serialize the contents of our model as XMI to our file on disk (i.e., ExtendedPO2.uml). To save a model using the UML editor, follow these steps:
- Select the File > Save menu item.
It’s that simple. Programmatically, we have a bit more work to do because so far, we’ve been creating our model in a vacuum, i.e. without a containing resource. The code snippet below shows a method that saves a specified package to a resource with a specified URI.
Here we create a resource set and a resource with the specified URI, add the package to the resource’s contents , and ask the resource to save itself using the default options. If an exception occurs, we notify the user via our handy utility method.
The above example uses the UMLResourcesUtil.init(...) API introduced in UML2 4.0.0 for Juno. As commented in the code snippet, this is not required in code running in the Eclipse Platform run-time, as in that case these registrations are discovered automatically from extension points.
OK, let’s see this method in action. For example, we could save the ‘epo2’ model to a resource with URI ‘ExtendedPO2.uml’ (relative to a URI passed in as an argument) as follows:
The UMLResource.FILE_EXTENSION constant represents the file extension for UML resources (.uml). Note that the UMLResource interface contains a number of constants that you will find useful when working with UML resources.
Congratulations! If you’ve made it this far, you’ve successfully created a simple model programmatically and/or using the UML editor. There’s a whole lot more that could be said, but the purpose of this article was just to get you started. Stay tuned for more articles on how to develop tools with UML2.

For more information on UML2, visit the home page or join a discussion in the forum.
The Papyrus Project provides graphical support for UML, SysML and MARTE models. See the Papyrus User Guide for further information.
[1] F. Budinsky, D. Steinberg, E. Merks, R. Ellersick, and T. J. Grose. Eclipse Modeling Framework. Pearson Education, Inc., Boston, MA, 2003.
Eclipse IDE. Download; Learn More; Documentation; Getting Started / Support; How to Contribute; IDE and Tools; Newcomer Forum; Search. Toggle navigation. Home; Marketplace; Free UML for Eclipse (3) Free UML for Eclipse (3) Search. 1478 Solutions and counting. Search. Advanced Search. Status. License Type. Spotlight. UMLet - UML Tool for Fast UML Diagrams. 701. 126. Install. Drag to Install. Download Eclipse UML Generators. Marketplace: Eclipse UML Generators 0.9.0 (Incubation) Update Sites: Eclipse UML Generators 0.9.0 (Incubation): Eclipse UML Generators 1.0.0RC5: Project Links. Wiki; Website; Documentation; Marketplace; Related Projects. Related Projects: Model Development Tools (MDT) Eclipse MDT UML2 ; Model To Text (M2T) Eclipse Acceleo; Eclipse Modeling Project. Eclipse. UML Designer is a graphical tool to edit and vizualize UML 2.5 models. It uses the standard UML2 metamodel provided by Eclipse Foundation and it implements the following generic UML diagrams: Package Hierarchy Class Diagam Component Diagram Composite Structure Diagram Deployment Diagram Use Case Diagram Activity Diagram State Machine Sequence Diagram Profile Diagra download a bundle, in this case UML designer is already installed, just unzip and run the umldesigner executable. get UML Designer in an existing Eclipse from the marketplace or from an update-site. If you decide to install this way, during the installation if you get the security warning saying that the authenticity or validity of the software can't be established, just click OK. Pay.
Free UML for Eclipse Eclipse Plugins, Bundles and
- AmaterasUML is an Eclipse plug-in for drawing UML which supports class-diagram, sequence-diagram and usecase-diagram. And it also provides Java support such as importing class/interfaces from Eclipse workspace and exporting class-diagram to Java source code
- Ce tutoriel décrit l'installation dans Eclipse du plugin UML DESIGNER. Il présente également comment réaliser un premier diagramme de classes avec ce produit et générer le squelette Java correspondant. Pour réagir au contenu de cet article, un espace de dialogue vous est proposé sur le forum 13 commentaires. Article lu fois. L'auteur. Marc Autran. L'article. Publié le 25 mai 2015.
- Packages Downloads. If you are interested in a specific package release of Eclipse Papyrus, you may find it directly in Eclipse Papyrus Packages Downloads. Update Sites Setup Eclipse Papyrus with Update Sites. The most basic procedure for installing Eclipse Papyrus consists in installing the Eclipse Modeling Package for your own platform
- Eclipse Papyrus relatives. Many technologies complement, extend or use Papyrus. Following are key ones: Papyrus RT for Real-time Systems modeling. Papyrus for Robotics: a Papyrus-based modeling environment dedicated to robotics. Papyrus PolarSys Solution: Eclipse Papyrus packaged as a PolarSys Solution. Eclipse UML Profiles Repository
- eclipse plugin uml free download. Eclipse Tomcat Plugin The Eclipse Tomcat Plugin provides simple integration of a tomcat servlet container for the develop
- Downloads for UML2 are published on the MDT downloads site.. Download Eclipse MDT UML2. Update Sites
Eclipse UML Generators projects
- eclipse uml free download. Modelio - Modeling environment (UML) Modelio is an open source modeling environment tool providing support for the latest standards (UML
- Eclipse Modeling Tools. 439 MB ; 10,386 DOWNLOADS; The Modeling package provides tools and runtimes for building model-based applications. You can use it to graphically design domain models, to leverage those models at design time by creating and editing dynamic instances, to collaborate via Eclipse's team support with facilities for comparing and merging models and model instances.
- Download. Below is the information of the remote Eclipse Update Site for the current release, which requires JDK 8. For The ObjectAid UML Explorer is an Eclipse plug-in, so you need a Java Development Kit and the Eclipse Java IDE to use it. JDK 8.0 or higher. For ObjectAid 1.1.x, JDK 6.0 can still be used. Eclipse 3.6.x or higher with the JDT (Java Development Tooling) GEF (Graphical.
- Version 1.9 is also available from mirror site https://nms.kcl.ac.uk/kevin.lano/uml2web/ Documentation is available from the github repository (files umlrsds19.pdf.

UML Designer Eclipse Plugins, Bundles and Products
Eclipse_UML_Generators builds are stored in p2 repositories that are produced as part of the build process. This page provides an overview of the different repositories maintained by the UML Generators project, and their corresponding location and retention policy. Update Sites List. Note: Each update site is released in one variant per supported target platform (e.g. luna and mars). All. Eclipse UML2 is an EMF-based implementation of the UMLTM 2.x metamodel for the Eclipse platform The Eclipse Project Downloads. On this page you can find the latest builds produced by the Eclipse Project.To get started, run the program and go through the user and developer documentation provided in the help system or see the web-based help system.If you have problems installing or getting the workbench to run, check out the Eclipse Project FAQ, or try posting a question to the forum
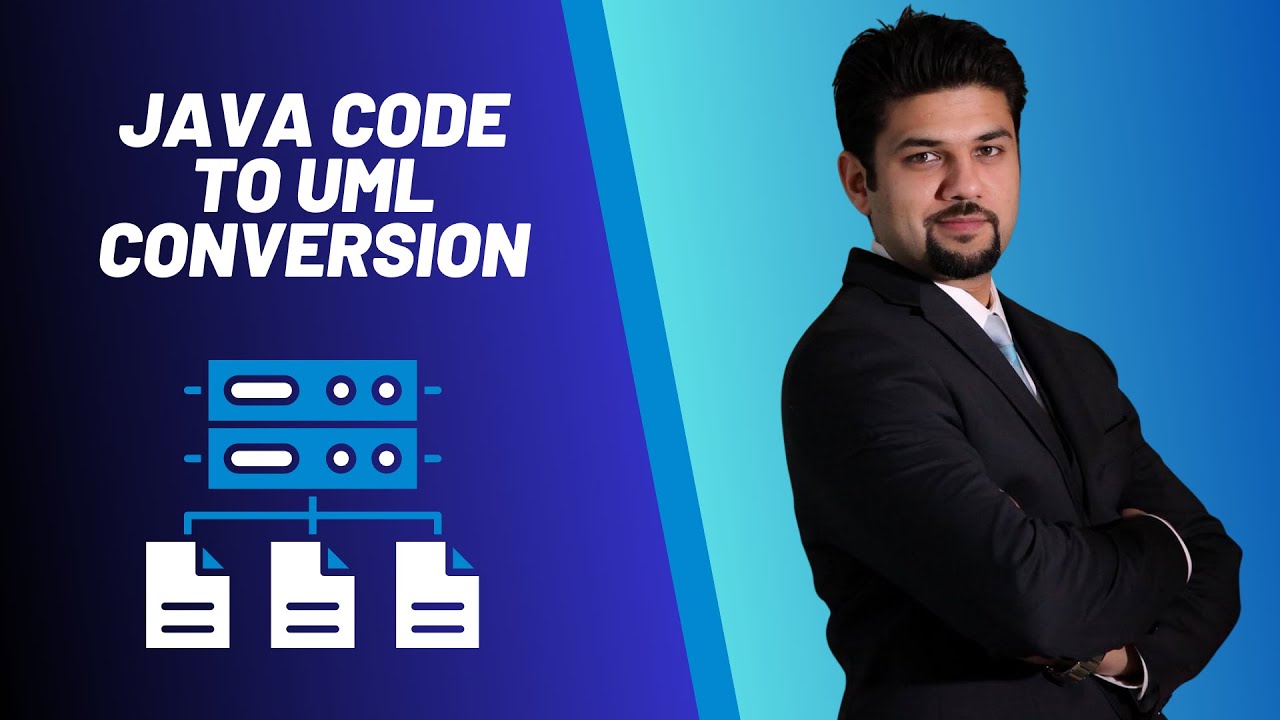
Download Eclipse UML Bot for free. An Eclipse plugin to view and manipulate UML diagrams. It does forward and reverse engineering of your java code into/from UML Each language pack zip contains 4 other zips (one for each of the language groups above). Unpack these zips into your Eclipse directory before starting Eclipse. These translations are based on UML2 2.0.0. The NLS translation fragment packs should work with all subsequent 2.0.x maintenance releases, with any new strings remaining untranslated Télécharger Eclipse : Un arsenal d'outils et de plugins dédiés au développement logiciel .746.000 reconnu programmes - 5.228.000 connu Recherches récentes. eclipse uml. eclipse uml. Recherches associées » auto generate uml c eclipse » slime uml eclipse » astah uml download_astah uml download » astah uml 6.7_astah uml download » astah uml for linux_astah uml download » eclipse 4.2.2_eclipse 4.2.2 download. Flutter Tutorial for Beginners - Build iOS and Android Apps with Google's Flutter & Dart - Duration: 3:22:19. Academind 847,441 view
Download - UML Designe
- UML plugin for Eclipse. Loading..
- UMLet is a free, open-source UML tool with a simple user interface: draw UML diagrams fast, build sequence and activity diagrams from plain text, export diagrams to eps, pdf, jpg, svg, and clipboard, share diagrams using Eclipse, and create new, custom UML elements. UMLet runs stand-alone or as Eclipse plug-in on Windows, OS X and Linux. Below the original, full-featured tool -- but also check.
- Summary. This article describes how to get started with the UML2 plug-ins for Eclipse. In particular, it gives an overview of how to create models (and their contents) both programmatically and by using the sample UML editor
- The ObjectAid UML Explorer is a freemium and lightweight tool that helps to visualize and generate Class and sequence diagrams straight from your java code. It is available as a free eclipse plugin in eclipse marketplace
- Download the UMLet zip file, unpack it, and start umlet.jar with a double-click. How to start UMLet as Eclipse-Plugin? Download the com.umlet.plugin*.jar file and copy it to Eclipse's plugin directory. To create a new, empty UMLet diagram in Eclipse, select a directory in the Eclipse resource navigator; select File/New/Other.. from the.
AmaterasUML Eclipse Plugins, Bundles and Products
- Eclipse IDE. Download; Learn More; Documentation; Getting Started / Support; How to Contribute; IDE and Tools; Newcomer Forum; Toggle navigation. Home; Marketplace; UML (40) UML (40) Search. 1477 Solutions and counting. Search. Advanced Search. Status. License Type. Spotlight. MetaModelAgent for Papyrus, IBM RSAD/RTE and HCL RTist. 2. 0. Install. Drag to Install! Drag to your running Eclipse.
- EclipseUML 3.2.0 Studio is Eclipse 3.2, Web Toolsand Callisto compliant EclipseUML 2.1.0 Studio is Eclipse 3.1 compliant; EclipseUML 2.0.0 Studio is Eclipse 3.0.2.
- The free UML tool UMLet lets you draw UML diagrams with a light-weight, pop-up-free user interface. It lets you draw diagram sketches fast; export diagrams to pdf, eps, jpg, gif, svg, bmp, png, and system clipboard; share diagrams using Eclipse 3+; and create your own custom graphical elements. New in version 14.2: Fixed pixel displacements on export; improved relations; embeddable pdf fonts
- Eclipse Modeling Tools can be useful as it contains a collection of Eclipse Modeling Project components, including EMF, GMF, MDT XSD/OCL/UML2, M2M, M2T, and EMFT elements. It includes a complete SDK, developer tools and source code. Please note that..
- Eclipse Uml Modeling Software UML Modeling for XML and SOA Design v.1.0 Development tools for modeling XML applications with UML , including UML profiles for XML Schema ans SOA
- Eclipse Uml Tutorial Software Eclipse UML Debugger v.1.0 A plug-in for Eclipse that allows users to debug programs using UML models in order to detect regions of divergence between the model and the program.
- IPGRAY : eclipse - How to download, install and use UML Designer plugin in eclipse
With this Eclipse UML tool, developers can perform visual modeling and other agile development activities within a unified Eclipse IDE platform. In this tutorial, you will walk through the steps required to integrate Visual Paradigm with Eclipse. The second part of the tutorial will demonstrate the automatic generation of Java code from UML class diagram. Preparation. In order to follow this. Papyrus UML, for instance, is based on UML2 Tool. There is a tool in the Marketplace that can do the above (UML Class, Package and Interaction). It's called ModelGoon. Last tried in July 2018. The full list is available at Eclipse marketplace Eclipse - Omondo - The Live UML Company : Release Policy: Studio Edition EclipseUML 2.2 Studio is Eclipse 3.5.2 Galileo compliant EclipseUML 2008 3.4.2 Studio is Eclipse 3.4.2 Ganymede compliant: EclipseUML 2008 3.3.0 Studio is Eclipse 3.3 and Europa compliant EclipseUML for Eclipse 3.5 Download : Home | Company | Product | Features. Eclipse - Omondo - The Live UML Company : EclipseUML 2.2 for Eclipse 3.5/3.5.2 download for Java and Java EE Modelers : On this page you can find our latest EclipseUML 2.2 Studio Edition release for Eclipse 3.5.2 SR2 Java and Java EE Modelers. Don't forget to modifiy your eclipse.ini file in order to avoid memory problem: -Xms40m-Xmx512m-XX:PermSize=512m. The test build below is currently.
The easiest way to get a version of Eclipse with all the UML plugins is to download the Eclipse Modeling Tools distribution from this page. share | follow | answered Jan 19 '12 at 6:36. ChrisH ChrisH. 4,638 23 23 silver badges 35 35 bronze badges. Yeah, I know. I installed Eclipse Modeling Tools but didn't work. - Omid Nazifi Jan 19 '12 at 10:35. add a comment | 3. If you only have. Eclipse UML Generators/New and Noteworthy/0.9.0 Jump to: navigation , search ---Navigation--- Main Page Community portal Current events Recent changes Random page Hel Eclipse Uml Editor, free eclipse uml editor freeware software downloads Eclipse Plugins Uml, free eclipse plugins uml freeware software downloads eclipse python uml free download. PyDev for Eclipse PyDev is a Python Development Environment (Python IDE plugin for Eclipse). It features an editor
graphical tooling to edit and visualize UML models. Download . Based on Sirius. Sirius is an Eclipse project which allows you to easily create your own graphical modeling workbench by leveraging the Eclipse Modeling technologies, including EMF and GMF. It provides a generic workbench for model-based architecture engineering that could be easily tailored to fit specific needs. Customizable and. Eclipse est un environnement de développement (IDE) historiquement destiné au langage Java, même si grâce à un système de plugins il peut également être utilisé avec d'autres langages de. I have mentioned very easy way to Create UML class diagram in eclipse so quickly. Enjoy ! Step by step easy guideline to create UML diagram in eclipse those.
Download File Objectaid Uml Explorer Eclipse Tutorials
Concevoir en UML sous Eclipse avec le plugin UML DESIGNER
- Provides an EMF-based implementation of the UML TM 2.5 metamodel for the Eclipse platform. Please refer to the org.eclipse.uml2.umlpackage documentation for API details
- Top 8 UML Modeling Tools Download When you are trying to design a system or program, you will need a standard way to visualize it which can be used to specify, construct and document the artifacts. A UML modeling tools open source can be used for this purpose
- La société Omondo propose EclipseUML qui est un plug-in permettant de réaliser des diagrammes UML dans Eclipse. EclipseUML propose une version gratuite avec le support des 11 diagrammes d'UML 2.0. Version utilisée dans cette section. Eclipse. 3.0.1. J2SE. 1.4.2_03. EclipseUML. 2.0.0. EclipseUML nécessite plusieurs plug-ins pour son fonctionnement avec Eclipse 3.0 : GEF (Graphical Editor.
- JS/UML is a plugin for the Eclipse IDE that reverse-engineers JavaScript code to a UML model which can then be used to generate documentation and other artifacts. Downloads: 19 This Week Last Update: 2016-05-10 See Projec
Eclipse Public License - v 2.0 THE ACCOMPANYING PROGRAM IS PROVIDED UNDER THE TERMS OF THIS ECLIPSE PUBLIC LICENSE (AGREEMENT). ANY USE, REPRODUCTION OR DISTRIBUTION OF THE PROGRAM CONSTITUTES RECIPIENT'S ACCEPTANCE OF THIS AGREEMENT. 1. DEFINITIONS Contribution means: a) in the case of the initial Contributor, the initial content Distributed under this Agreement, and b) in the case of. UMLet is a free, open-source UML tool with a simple user interface: draw UML diagrams fast, produce sequence and activity diagrams from plain text, export diagrams to eps, pdf, jpg, svg, and clipboard, share diagrams using Eclipse, and create new, custom UML elements. UMLet runs stand-alone or as Eclipse plug-in on Windows, OS X and Linux org.eclipse.uml2.uml Interface ElementImport All Superinterfaces: DirectedRelationship, Element, org.eclipse.emf.ecore.EModelElement, org.eclipse.emf.ecore.EObject.
UML2 is an EMF-based implementation of the Unified Modeling Language (UML) 2.x OMG metamodel for the Eclipse platform.. The objectives of the UML2 component are to provide a useable implementation of the UML metamodel to support the development of modeling tool org.eclipse.emf.common.util.EList<Dependency> getClientDependencies() Returns the value of the 'Client Dependency' reference list. The list contents are of type Dependency. Indicates the Dependencies that reference this NamedElement as a client. From package UML::CommonStructure. Returns: the value of the 'Client Dependency' reference list. See. extends org.eclipse.emf.ecore.EModelElement. A representation of the model object 'Element'. An Element is a constituent of a model. As such, it has the capability of owning other Elements. From package UML::CommonStructure. The following features are supported: Owned Comment; Owned Element; Owner; See Also: UMLPackage.getElement() Method Summary; boolean: addKeyword(java.lang.String keyword. Umbrello installer and portable packages for 32bit and 64 bit Windows are available at the KDE download mirror network. Umbrello on Windows is also part of the KDE on Windows distribution installed with the KDE-Installer. Please note that the KDE on Windows distribution does not contain the latest umbrello releases. Mac OS
Download ArgoUML 0.35.1 for Windows. Fast downloads of the latest free software! Click no Download Eclipse Papyrus. Update Sites: 4.x - Master (Main): 3.x - Oxygen Release (Main): 3.x - Oxygen Nightly (Main): 2.0.y - Neon Release (Main + Extra): 2.0.y - Neon Nightly (Main + Extra): 1.1.x - Mars Release (Main + Extra): 1.0.x - Luna Release (Main + Extra): Downloads: Eclipse Papyrus downloadable zips. Project Links. Documentation; Getting Started; Website; Wiki; Related Projects. To activate UML Lab after the trial period has ended, you will need a license key. You will get the key without delay after purchase and payment of a UML Lab license in our webshop or after registration for our Academic Program The Eclipse UML2 (Unified Modeling Language 2.x) project is an EMF-based implementation of the UML 2.x metamodel for the Eclipse platform. This implementation was previously compliant with the 2.2 version of the UML specification, for which newer versions, namely 2.3 and 2.4(.1), have been released by the Object Management Group (OMG) over the past couple of years. The source code for the UML2. slime uml eclipse Gratuit Télécharger logiciels à UpdateStar - Slime UML (SLIm Modelling Environment) is a slim,fast, and powerful UML plugin the Eclipse platform. It makes it simple to document Java code, reverse-engineer existing source code, andvisualise complex Java libraries, even
Eclipse Downloads The Eclipse Foundatio
Lisez sur 01netPro: Eclipse 3.6 Helios en images. Version : 2018-09 - 32 bits. Licence : Logiciel libre. Taille : 189.4 Mo. Configuration minimale : Windows 7/8/8.1/10 JVM. Date de sortie : 18/09. Eclipse 3.3 Support (This version can't work with Eclipse 3.2.x) Copy & Pase in the class diagram, the usecase diagram and the activity diagram. Brand new visual theme for diagrams. See details here about new features. 2007/04/22 - Version 1.2.2. Activity diagram is available. Connection routers which exclude BendpoinConnectionRouter are not.
eUML2 Studio is a powerful set of tools developed from scratch for Eclipse. These tools are designed specially for developers to put UML in action at the development level: ensure the software quality and reduce the development time Eclipse uml omondo Mettre eclipse en français - Forum - Programmation Désinstaller eclipse - Forum - Linux / Uni
Visual Paradigm enables you to integrate the visual modeling environment with Eclipse, providing full software development life cycle support.By designing your software system in Visual Paradigm, you can generate programming source code from class diagram to an Eclipse project.Also, you can reverse engineer your source code into class models in Visual Paradigm Download UML Diagram Maker for Windows to create UML and ERD diagrams for software engineers and designers In this video will be explained how to install the Papyrus plugin for UML Modelling in Eclipse. Next to installation of the Plugin, it will also be shown how.. C'est un outil UML avec une interface utilisateur simple : dessiner des diagrammes UML rapidement, produire des séquence et des diagrammes d'activité, du texte brut, l'exportation des diagrammes en EPS, PDF, JPG, SVG et presse-papiers, partagez vos diagrammes en utilisant Eclipse, et créer de nouveaux éléments. UMLet fonctionne de façon autonome ou comme plug-in d'Eclipse sous Windows.
Papyrus Dowloads - Eclipse
Objecteering/UML, premier atelier UMLTM (Unified Modeling Language) supportant la démarche MDATM de l'OMG (Object Management Group), regroupe les fonctions de gestion d'exigences, de modélisation UML avec vérification de cohérence en ligne, d'assistance méthodologique, de génération de documentation d'analyse et conception, d'automatisation de design patterns, de génération de code. org.eclipse.ocl.all.sdk (OCL, implémentation de référence du langage OCL de l'OMG, utilisé entre autres pour définir des contraintes et des requêtes sur des modèles EMF) org.eclipse.uml2.sdk (UML, implémentation du méta-modèle UML réalisé avec EMF et outillage associé) org.eclipse.xsd.sdk (XSD, outillage de manipulation de fichiers XSD) UML. Le projet Eclipse Modeling héberge une.
Eclipse 3.4 + Eclipse UML zip file, which is a Stand Alone Eclipse zip file which contains Eclipse 3.3, EclipseUML 2008 Edition, EMF, GEF and UML2. 1. To install the EclipseUML zip file, you need to select your Eclipse workspace. Select your Eclipse folder which contains your Eclipse 3.4 installation. You should have these files in your folder: 3 Looking for UML plugin for your most favorable Eclipse IDE? Visual Paradigm is your only choice. Now, you can do both coding and system modeling right inside the Eclipse without switching around two applications. Seeing is believing! This plugin provide all the features you need for application development that you can't found elsewhere. Search no more, download and try it Now Release Build: R200406281334 June 28, 2004 . These downloads are provided under the Eclipse.org Software User Agreement.. To view the build notes for this build click here. To view the test results and compile logs for this build click here. To view the map file entries for this build click click here.. Click here for instructions on how to verify the integrity of your downloads Ajout du plug-in UML dans Eclipse. La modélisation de l'application de gestion est réalisée avec un logiciel intégré dans Eclipse. Il existe plusieurs plug-ins UML pour Eclipse, certains gratuits d'autres payants. Le choix d'un plug-in est une question d'habitudes, de coût, et même de goût Afin de rester dans un esprit open source, le plug-in Papyrus, qui est totalement. Visual Paradigm SDE for Eclipse is a UML CASE tool/plug-in tightly integrated with Eclipse.This UML tool supports full software lifecycle - analysis, design, implementation, testing and deployment. This OO modeling tool helps you build quality applications faster, better and cheaper. You can draw all types of UML diagrams in Eclipse, reverse engineer Java code to class diagrams, generate Java.
Papyrus est open source (licence EPL) et basé sur la technologie Eclipse. Il s'agit d'une version graphique du plugin UML2 de Eclipse. Objecteering/UML, premier atelier UMLTM (Unified Modeling Language) supportant la démarche MDATM de l'OMG (Object Management Group), regroupe les fonctions de gestion d'exigences, de modélisation UML avec vérification de cohérence en ligne, d. The tool can works online, as Eclipse plugin, and also stand-alone command-line Jar. Download link: https://cruise.eecs.uottawa.ca/umple/ 7) Visual Paradigm. Visual Paradigm is a software design tool which is tailored for engine software projects. This UML tool helps the software development team to a model business information system and development processes. Features: It offers support for. Almost 2 years late, but hey. I came across this question looking for other UML tools for Eclipse and figured that I might be able to help. I'm glad I posted it. - Thomas Owens Sep 2 '11 at 19:38. Well, the other (minor) one is that you can create the diagrams from the outline view only and not from the editor. But still the best tool by far - kostja Sep 2 '11 at 19:40. Yeah, there are. You could also give the netbeans UML modeller a try. I have used it to generate javacode that I used in my eclipse projects. You can even import eclipse projects in netbeans and keep the eclipse settings synced with the netbeans project settings. I tried several UML modellers for eclipse and wasn't satisfied with them. They were either unstable.
Objectaid Uml Eclipse
Eclipse Papyrus - The Eclipse Foundatio
What is the version of Eclipse that can be used to draw the UML diagrams. That is what is the version of Java with which the tools to draw UML diagrams comes along with it. Thanks. eclipse uml. share | follow | edited Jan 20 '14 at 14:25. informatik01. 14.8k 8 8 gold badges 66 66 silver badges 100 100 bronze badges. asked Mar 26 '12 at 9:34. user1227433 user1227433. 835 4 4 gold badges 9 9 si et je souhaite creer les differents diagramme, scenario, etc..en uml a partir de ce script sql. j utilise win design 6.5.1, et j ai l impression que ce n est pas possible. quelqu un a deja fait ? merci ! Répondre avec citation 0 0. 17/03/2009, 18h43 #6. Vlade. Inactif Crée classe diagramme à partir d'un script sql Bonjour Vidoluc, Il faut utiliser le plugin dali d'Eclipse afin de récréer Be warned that free version of Omondo UML Eclipse Plugin does not allow created UML diagrams to be shared! This implies that if you ever reinstall your Eclipse, you will no longer be able to open the diagrams you created earlier! First of all, we need to download the plugin Eclipse - Omondo - The Live UML Company : UML 2.2 Diagrams : Maven Modeling Cycle: Oracle Enterprise Pack Eclipse 11g : Dynamic Navigation: Live code and model synchronization: UML Profile diagram: Persistence Development: Reverse Engineering Architecture : Database Reverse engineering Java or UML or both ? XMI Editor: Reverse Legacy Jar File: Powered by Eclipse . EclipseUML architecture is.
eclipse plugin uml free download - SourceForg
Eclipse UML Generators provides components that automatically bridge the gap between UML models and source code. Either by extracting data from UML models (and UML profiles or decoration models) to produce source code or by reverse-engineering source code to produce UML models. Code generation uses Acceleo which is a pragmatic implementation of the Object Management Group (OMG) MOF Model to. Code generation creates and updates source files in a Java project from UML models.You can select to update the whole project, package(s) and class(es) from Visual Paradigm to Eclipse.Before updating source files, you must open the UML project from the Java project. Project Based Code Generatio Eclipse IDE. Download; Learn More; Documentation; Getting Started / Support; How to Contribute; IDE and Tools; Newcomer Forum; Search. Toggle navigation. Breadcrumbs. Home; Contribute; Source code ; index: org.eclipse.umlgen.git: Eclipse UML Generators: about summary refs log tree commit diff stats: Age Commit message Author Files Lines; 2019-01-05: Updated auto-coded sources and added missing. Eclipse Uml Linux software, free downloads. Eclipse Uml Linux shareware, freeware, demos: MaintainJ Plugin by MaintainJ Inc, Eclipse PDT for Linux by Eclipse Foundation, MDG Integration for Eclipse by Sparx Systems etc.. Introduction Introduction à UML UML et l'OMG OMG = Object Management Group (www.omg.org) : Fondé en 1989 pour standardiser et promouvoir l'objet Version 1.0 d'UML (Unified Modeling Language) en janvier 1997 Version 2.5 en octobre 2012 Définition d'UML selon l'OMG : Langage visuel dédié à la spécification, la construction et l
Welcome to UML Modeling by Obeo Network. UML Designer provides a set of common diagrams to work with UML 2.5 models. The intent is to provide an easy way to make the transition from UML to domain specific modeling. This way users can continue to manipulate legacy UML models and start working with DSL.Users can even re-use the provided representations and work in a total transparence on both. EclipseUML 3.2.0 Studio is Eclipse 3.2, Web Toolsand Callisto compliant; EclipseUML 2.1.0 Free is Eclipse 3.1 compliant. EclipseUML 2.0.0 Free is Eclipse 3.0.2 compliant. EclipseUML 1.2.1 Free is Eclipse 2.1.2 compliant. EclipseUML 1.2.0 Free is Eclipse 2.0.2 and WSAD 5 compliant. Latest Download: EclipseUML 2.1.0.20061006 Free: EclipseUML 3.2.0.20061003 Studio Last update Wed July 12 16:28:11. J'aimerais installer le plug-in Modeling SDK pour créer des diagrammes en UML 2 dans mon Eclipse Juno for JEE developers, mais je ne trouve pas le l'update-site à ajouter, dans la boite de dialogue qu'affiche Eclipse. Quelqu'un saurait-il m'indiquer où le trouver ? Merci pour votre aide. Répondre avec citation 0 0. 26/02/2013, 10h46 #2. Gueritarish. Expert confirmé Salut, Dans ton.
These downloads are provided under the Eclipse.org Software User Agreement. To view The Eclipse driver used in this build was eclipse-SDK-R-3.1.1-200509290840-linux-gtk.tar.gz - for details on this driver or to download it for your platform, check out its build page. The EMF build was emf-sdo-xsd-SDK-2.1.1.zip from this build page. SDK: This download is the developer's SDK. It contains the. Dans le cadre de l'ingénierie dirigée par les modèles, ce tutoriel vous présente les étapes à suivre pour la création et l'utilisation d'un profil UML en utilisant l'outil Papyrus. Ce dernier est un outil de modélisation sous Eclipse, sa description ainsi que la procédure d'installation sont aussi traitées dans ce tutoriel In this video, we demonstrate UML modeling using a free plugin called Papyrus on Eclipse. The video describes a class diagram example. The link of Papyrus: ht..
Eclipse MDT UML2 projects
Create a new UML model & UML project. To create a new UML project, enable the Modeling Perspective. Then click on the wizard shortcuts at the top-right of the Eclipse Workbench. Select New UML Project. You can choose specific name for the project and the root element for the project on the next pages Download UMLet for free. UMLet is a free, open-source UML tool with a simple user interface: draw UML diagrams fast, produce sequence and activity diagrams from plain text, export diagrams to eps, pdf, jpg, svg, and clipboard, share diagrams using Eclipse, and create new, custom UML elements The UML Designer developers are hanging out on #eclipse-modeling on Mattermost, feel free to come and chat. Bug Tracker Review and create GitHub issues for bug reporting and any feature request Eclipse est un environnement de développement intégré pour des applications open source et multi-plateformes. Cela fonctionne principalement comme plateforme de programmation, et il peut compiler et déboguer beaucoup de langues de programmation: bien qu'ils soit plus connu pour la programmation dans Java, sa modularité te permet de l'utiliser pour la programmation en C, Pytho, et ainsi. UMLet is an open-source UML tool with a simple user interface: draw UML diagrams fast, export diagrams to eps, pdf, jpg, svg, and clipboard, share diagrams using Eclipse, and create new, custom UML elements. Please check out the Wiki for frequently asked question
eclipse uml free download - SourceForg
A team of Eclipse experts. Strategic member of the Eclipse Fondation, Obeo helps you to develop state-of-the-art Eclipse-based tooling. Our experts, including 20 Eclipse committers, master the development process of high-quality Eclipse plug-ins. We provide a wide range of services: training, consulting, custom development and support & maintenance Downloads Downloads; Source Code Repository; Source Code Repository; Wiki Wiki; About this Project. UML was started in November 2009, is owned by Javier Ortiz, and has 194 members. » Join This Project; NetBeans.org. Join; News.
Eclipse Packages The Eclipse Foundation - home to a
UML Designer provides a set of common diagrams to work with UML 2.5 models. The intent is to provide an easy way to make the transition from UML to domain specific modeling. This way users can continue to manipulate legacy UML models and start working with DSL. Users can even re-use the provided representations and work in a total transparence on both UML and DSL models at the same time Free Downloads DBMS Repository Scripts User Security Key. All Resources > UML Tutorial; Community; Login; Products; MDG; MDG Link; Eclipse ; Eclipse - MDG Link. Light and agile bridge linking Enterprise Architect and Eclipse The Model Driven Generation (MDG) Link for Eclipse provides an integrated solution for users of the Professional and Corporate versions of Enterprise Architect and Eclipse. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58.
Download - ObjectAid UML Explore
The Eclipse open source tools project spearheaded by IBM and the Object Management Group's Unified Modeling Language (UML) 2.0 both are taking steps forward Download Support keyboard_arrow_right. Install ObjectAid Obtain a License Uninstall ObjectAid Resellers FAQ Contact The ObjectAid UML Explorer. The ObjectAid UML Explorer is an agile and lightweight code visualization tool for the Eclipse IDE. It uses the UML notation to show a graphical representation of existing Java code that is as accurate and up-to-date as your text editor, while being. It is based on UMLet (which is available as stand-alone tool or Eclipse plugin), and shares its fast, text-based way of drawing UML sketches. Main features: install-free web app; save diagrams in browser storage; support for many UML diagram types; simple, markup-based UML element modifications; png export. Start UMLetino NOW! (JavaScript and Cookies required) Follow @twumlet Tweet. UML Lab est un logiciel de Shareware dans la catégorie Divers développé par Yatta Solutions GmbH. La dernière version de UML Lab est actuellement inconnue. Au départ, il a été ajouté à notre base de données sur 03/09/2010. UML Lab s'exécute sur les systèmes d'exploitation suivants : Windows Gratis slime uml eclipse Hämta programvara UpdateStar - Slime UML (SLIm Modelling Environment) is a slim,fast, and powerful UML plugin the Eclipse platform. It makes it simple to document Java code, reverse-engineer existing source code, andvisualise complex Java libraries, even